# Image API
# Filter
Filters are used to transform images.
Color.ImageToURL(url, ImageFilter.grayscale(100), (newFilterImageUrl) => {
console.log(newFilterImageUrl) // return datauri
})
2
3
The above function passes the image url as a parameter and returns the image url (newFilterImageUrl) with the grayscale filter applied as datauri.
# Create Filter
Filters can be combined.
# single filter
ImageFilter.grayscale(100) // single filter
# multi filter
ImageFilter.multi(
ImageFilter.grayscale(100),
ImageFilter.sepia(100)
)
2
3
4
You can use it simply.
ImageFilter.multi(
['grayscale', 100],
['sepia', 100]
)
2
3
4
5
# Make Filter
The Filter consists of simple functions.
ImageFilter.myfilter = function () {
return function (bitmap) {
// bitmap.pixels
// bitmap.width
// bitmap.height
// Create a new Bitmap object using a Bitmap object.
// Or you can transform the old bitmap object and return it again.
return bitmap
}
}
2
3
4
5
6
7
8
9
10
11
12
The following sample is a filter that slightly increases the overall brightness of the image.
As the RGB color standard becomes brighter, it is better to add RGB one more.
ImageFilter.brightness = function () {
return ImageFilter.pack((pixels, i) => {
pixels[i] += 1; // red
pixels[i + 1] += 1; // green
pixels[i + 2] += 1; // blue
})
}
2
3
4
5
6
7
TIP
Handling bitmap objects is complicated, so there are a few utility functions that you can use to easily handle rgb.
# Filter Util
# ImageFilter.pack
Create a Loop to easily control RGB colors.
ImageFilter.pack((pixels, i) => {
pixels[i] += 1; // red
pixels[i + 1] += 1; // green
pixels[i + 2] += 1; // blue
})
2
3
4
5
# ImageFilter.convolution
Higher-order filter functions
// blur
F.convolution([
1/9, 1/9, 1/9,
1/9, 1/9, 1/9,
1/9, 1/9, 1/9
]);
2
3
4
5
6
TIP
https://en.wikipedia.org/wiki/Kernel_(image_processing)
# ImageFilter.multi
It combines several filter functions into one filter function.
ImageFilter.multi(
'blur',
'grayscale',
'sharpen',
['blur', 3],
function (bitmap) {
return bitmap
}
);
2
3
4
5
6
7
8
9
# ImageFilter.merge
It combines array type filters similar to multi to create filter function.
ImageFilter.merge([
'blur',
'grayscale',
'sharpen',
['blur', 3],
function (bitmap) {
return bitmap
}
]);
2
3
4
5
6
7
8
9
# ImageFilter.counter
A filter function that creates a run count for a specific filter.
ImageFilter.counter('grayscale', 10) // filter function to execute grayscale 10 times
# Filter List
# GrayScale
ImageFilter.grayscale(amount) // amount is 0..100
# Contrast
ImageFilter.contrast(amount) // amount is -100..100
# Saturation
ImageFilter.saturation(amount) // amount is 0..100
# Brightness
ImageFilter.brightness(amount) // amount is 0..100
# Noise
ImageFilter.noise(amount) // amount is 1..100
# Gradient
ImageFilter.gradient(colors, scale) // scale is default value 256
Please refer to Gradient
# Sepia
ImageFilter.sepia(amount) // amount is -100..100
# Negative
ImageFilter.negative(amount) // amount is 0..100
# Threshold
ImageFilter.threshold(scale, amount) // scale is 0..255, amount is -100..100
# Hue
ImageFilter.hue(amount) // amount is 0..360
# Shade
ImageFilter.shade(r, g, b) // r,g,b is 0..10 , step = 0.1
# Invert
ImageFilter.invert()
# Sharpen
ImageFilter.sharpen(amount)
# Emboss
ImageFilter.emboss(amount) // amount is 0..50, step = 0.1
# Blur
ImageFilter.blur(radius) // radius is 3..100
# Stack Blur
ImageFilter['stack-blur'](radius) // radius is 3..100
TIP
https://github.com/flozz/StackBlur
# Motion Blur
ImageFilter['motion-blur'](amount) // amount is 0..100
# Laplacian
ImageFilter.laplacian(amount) // amount is 0..100
# Sobel
ImageFilter.sobel(amount) // amount is 0..100
# Histogram
A histogram of a specific image can be obtained as an image.
Color.ImageToHistogram(this.imageSrc, (url) => {
this.filteredImageSrc = url;
})
2
3
Basically, only the histogram with the contrast ratio is imaged.
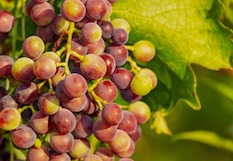
R, G, and B regions.
Color.ImageToHistogram(this.imageSrc, (url) => {
this.filteredImageSrc = url;
}, { red: true, green: false, blue: true })
2
3
# Get histogram list
Color.histogram(url, function ( { black, red, green, blue } ) {
// arr is histogram list
})
2
3
# Palette
You can pick the color you use the most in the image.
Color.ImageToRGB(url, { maxWidth: 100 }, (results) => {
// Extract color by colorCount
this.paletteColors = Color.palette(results, colorCount).map(color => {
const contrast = Color.contrast(color)
return { color, contrast, textColor: contrast >= 128 ? 'black' : 'white' }
}).sort((a, b) => { // sort by contrast
if (a.contrast === b.contrast) return 0
return a.contrast > b.contrast ? 1 : -1;
})
})
2
3
4
5
6
7
8
9
10
11
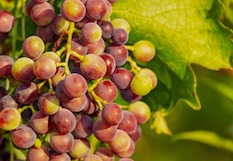
TIP
Internally, use the [K-means] (https://en.wikipedia.org/wiki/K-means_clustering) algorithm to extract the final color.
This is useful for extracting colors from a pixel image.
← Image